Error Call to a Member Function getCollectionParentId() on Null: Understanding and Resolving the Issue
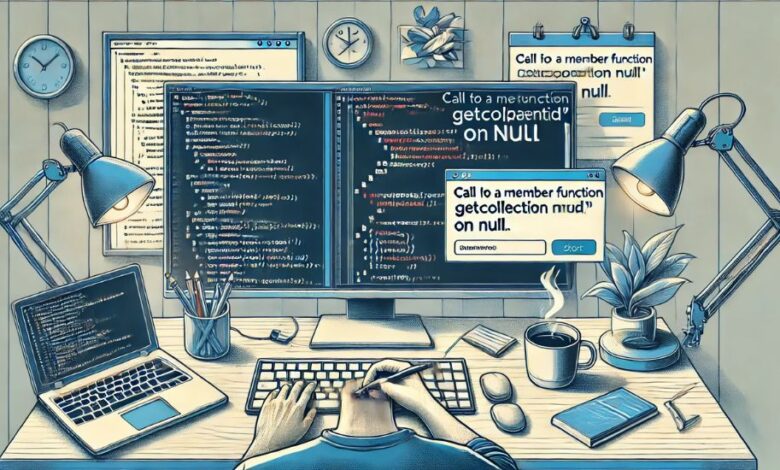
When developing web applications, especially with PHP and frameworks like Magento or custom CMS platforms, encountering errors is inevitable. One such common and frustrating error is “Error Call to a Member Function getCollectionParentId() on Null”. This issue can disrupt workflows and compromise the functionality of your application. Understanding the causes, impacts, and resolutions of this error is essential for smooth development and efficient debugging.
This article provides a comprehensive look into “Error Call to a Member Function getCollectionParentId() on Null”, explaining its meaning, common causes, and detailed troubleshooting steps. We will also share best practices to prevent this error in the future.
What is “Error Call to a Member Function getCollectionParentId() on Null”?
The error “Call to a member function getCollectionParentId() on null” occurs in PHP when the code attempts to execute the getCollectionParentId()
method on an object that is null
. In simple terms, it means the object you are trying to interact with doesn’t exist or has not been initialized properly.
This error is prevalent in systems where dynamic data or objects are retrieved from external sources, such as databases or APIs. If the expected object isn’t created successfully, PHP throws this fatal error, halting the script’s execution.
Common Causes of the Error
1. Uninitialized Object
The most common reason for this error is attempting to use an object that hasn’t been properly instantiated. If the object is not created or initialized, calling any method on it will result in a null reference error.
2. Database Query Returns Null
If your application relies on a database query to fetch the object, a failed or empty query can result in a null object. For instance, if no record matches the query criteria, the expected object won’t exist.
3. Incorrect Variable Assignment
Sometimes, coding mistakes such as overwriting the object variable with null
or another incompatible value can cause this issue. This might happen during complex operations where multiple variables are interdependent.
4. Logical Flaws in Code
Errors in conditional logic or function calls can lead to scenarios where the object is expected but not actually created. For example, a missing if
condition might bypass the instantiation process.
How to Troubleshoot “Error Call to a Member Function getCollectionParentId() on Null”
Step 1: Check Object Initialization
Ensure the object is initialized before calling getCollectionParentId()
. For example:
if ($object !== null) {
$parentId = $object->getCollectionParentId();
} else {
echo "Object is not initialized.";
}
This simple check ensures that the object exists before attempting to use it.
Step 2: Inspect Database Queries
If the object is fetched from a database, verify the query:
$object = $database->fetchObject($query);
if ($object === null) {
echo "No matching record found in the database.";
}
Make sure the query returns valid data and handles cases where no results are found.
Step 3: Review Variable Assignments
Double-check your code to ensure the variable holding the object isn’t accidentally overwritten. Use debugging tools or add log statements to track variable values.
Step 4: Enable Error Logging
Turn on detailed error logging to identify the exact location and cause of the error. Use PHP’s error logging functions:
error_log("Object initialization failed in file: " . __FILE__);
Preventing “Error Call to a Member Function getCollectionParentId() on Null”
1. Always Initialize Objects
Ensure all objects are properly initialized with default values or constructors. For example:
$object = new MyObject();
2. Validate Database Queries
Design your queries to handle cases where no results are found. Implement fallback mechanisms, such as default values or placeholder objects.
3. Implement Error Handling
Use try-catch blocks to handle exceptions and provide meaningful error messages.
try {
$parentId = $object->getCollectionParentId();
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
4. Review Application Logic
Conduct regular code reviews to identify and fix logical errors that might prevent proper object initialization.
Real-Life Examples of the Error
Example 1: Fetching Data from a CMS
A developer might write code to retrieve a page’s parent ID using a CMS function:
$page = $cms->getPage($pageId);
$parentId = $page->getCollectionParentId();
If $cms->getPage()
fails to return a valid object, the error occurs. Adding a null check resolves the issue:
if ($page !== null) {
$parentId = $page->getCollectionParentId();
}
Example 2: Querying an E-commerce Database
In an e-commerce platform, the code may try to fetch a product category’s parent ID:
$category = $productModel->getCategory($categoryId);
$parentId = $category->getCollectionParentId();
If $productModel->getCategory()
returns null, the solution is to validate the category object:
if ($category !== null) {
$parentId = $category->getCollectionParentId();
}
} else {
echo "Category not found.";
}
Conclusion
The error “Call to a member function getCollectionParentId() on null” may seem daunting at first, but with proper understanding and debugging techniques, it can be effectively resolved. By focusing on object initialization, error handling, and application logic, developers can prevent this issue from recurring.
If you’re facing this error in your project, following the steps outlined above will help you diagnose and resolve it efficiently. For more in-depth tutorials and troubleshooting tips, visit our blog Mating Press to stay updated with the latest programming insights.